Java backend developer skills are critical for creating robust, scalable, and secure server-side applications.
As businesses increasingly rely on dynamic, data-driven platforms, a skilled Java developer is in high demand.
From knowledge of frameworks and databases to understanding performance optimization processes, mastering Java backend technologies ensures that specialists are prepared to build the most effective systems.
What are Java backend skills?
Java backend skills are the core competencies that a specialist needs to effectively build and maintain the server-side architecture of applications using the Java programming language.
These abilities focus on various aspects of backend development, which include handling data, ensuring system performance, and creating secure, scalable solutions.
Create your professional Resume in 10 minutes for FREE
Build My Resume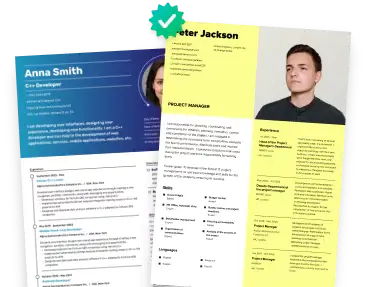
Essential Java backend developer skills
1. Core Java Backend Programming Skills
It refers to the foundational concepts and features of the Java language, including syntax, data types, operators, control flow, and fundamental libraries.
This also involves understanding key programming concepts such as classes, objects, inheritance, polymorphism, and abstraction.
- Learning time
- Typically ranges from 3 to 6 months, depending on prior programming experience and the time you can dedicate to practice. It’s best learned progressively, with a focus on hands-on coding.
How to learn:
- Focus on Java syntax, control structures, and simple object-oriented principles.
- "Head First Java" by Kathy Sierra & Bert Bates (book)
- Codecademy’s Java Course (interactive course)
- Java Programming and Software Engineering Fundamentals on Coursera (specialization)
- Build small projects or solve problems on coding platforms to reinforce what you’ve learned.
- LeetCode or HackerRank (practice problems)
- Exercism.io (practice with mentorship)
- Read the official Java API documentation to understand how the standard libraries and frameworks work.
- Oracle’s Official Java Documentation (website)
- Focus on Java syntax, control structures, and simple object-oriented principles.
2. Object-Oriented Programming (OOP)
OOP is a programming paradigm that structures code around objects and their interactions. It involves key principles like inheritance, encapsulation, abstraction, and polymorphism, which are essential for designing scalable and maintainable Java applications.
- Learning time
- Typically takes 2 to 4 months, depending on the depth and practice involved. Understanding these principles is critical for writing reusable and clean code.
How to learn:
- Study the principles of OOP and practice applying them in Java programs.
- "Java: A Beginner's Guide" by Herbert Schildt (book)
- Udemy’s Java Masterclass (video course)
- Work on small projects focusing on OOP concepts (e.g., creating a library management system).
- W3Schools OOP Tutorials (free tutorials)
- Watch OOP-focused content on YouTube for hands-on examples and explanations.
- Core Java OOP Tutorials by Telusko (YouTube)
- Study the principles of OOP and practice applying them in Java programs.
3. Multithreading and Concurrency
This involves creating and managing multiple threads in Java to perform tasks concurrently. It is essential for building high-performance applications that handle multiple operations simultaneously.
- Learning time
- Takes 2 to 3 months to learn the basics. Advanced multithreading can take longer as it requires hands-on experience and understanding of concurrent programming.
How to learn:
- Learn about Java's threading model and key classes like
Thread
,Runnable
, andExecutorService
.- "Java Concurrency in Practice" by Brian Goetz (book)
- Oracle's Java Concurrency Tutorial (online guide)
- Practice writing simple multithreaded programs and gradually move to more complex use cases.
- GeeksforGeeks Java Multithreading (tutorials)
- Experiment with thread-safe collections and synchronization techniques.
- HackerRank or CodeSignal (coding challenges)
- Learn about Java's threading model and key classes like
4. Exception Handling
This allows developers to manage and resolve runtime errors gracefully, ensuring application stability and a better user experience.
- Learning time
- Mastering exception handling concepts can take 1 to 2 months, with ongoing practice required to identify and handle edge cases.
How to learn:
- Study Java's exception hierarchy and understand how to use
try
,catch
,finally
,throw
, andthrows
.- GeeksforGeeks Java Exception Handling (tutorials)
- Analyze examples of custom exceptions and error-handling strategies.
- "Effective Java" by Joshua Bloch (book)
- Practice debugging and handling exceptions in real-world coding scenarios.
- Exercism.io Java Track (practice)
- Study Java's exception hierarchy and understand how to use
5. Java Collections Framework
It provides a set of classes and interfaces for managing data efficiently, such as lists, sets, maps, and queues.
- Learning time
- Takes around 1 to 2 months to learn and practice the core data structures and their use cases.
How to learn:
- Familiarize yourself with the different types of collections and their purposes.
- GeeksforGeeks Java Collections (tutorials)
- "Java: The Complete Reference" by Herbert Schildt (book)
- Practice solving coding problems related to collections.
- HackerRank Data Structures Challenges (platform)
- Study real-world use cases of collections in backend applications.
- Medium Articles on Java Collections (blogs)
- Familiarize yourself with the different types of collections and their purposes.
6. Java Frameworks
Java frameworks, such as Spring and Hibernate, simplify backend development by providing prebuilt tools and patterns for common tasks like dependency injection, ORM, and REST API.
- Learning time
- Can take 2 to 4 months, depending on the complexity and prior experience with Java.
How to learn:
- Start with beginner-friendly guides to popular frameworks.
- Spring Framework Documentation (website)
- Baeldung Spring Tutorials (website)
- Build small projects like a REST API or a CRUD application using the framework.
- "Spring in Action" by Craig Walls (book)
- Take online courses focusing on Java frameworks.
- Spring Boot Basics on Pluralsight (video course)
- Start with beginner-friendly guides to popular frameworks.
7. Database Management
This involves understanding how to interact with databases, store and retrieve data, and optimize database queries.
- Learning time
- Can take 2 to 3 months to learn basic database operations and integrations with Java.
How to learn:
- Study relational and non-relational database concepts.
- W3Schools SQL Tutorial (online guide)
- MongoDB University (NoSQL course)
- Practice database integration with Java using JDBC or an ORM framework.
- Official JDBC Guide (documentation)
- Build a project that integrates a database, like a simple inventory management system.
- Udemy SQL & Database Courses (video course)
- Study relational and non-relational database concepts.
8. API Development (RESTful Services)
API development involves creating interfaces that allow applications to communicate with each other. RESTful services are a common standard for building scalable, lightweight, and maintainable APIs.
- Learning time
- Takes around 2 to 3 months to understand the principles of API design and implementation using Java.
How to learn:
- Learn the basics of REST architecture and HTTP methods (GET, POST, PUT, DELETE).
- RESTful Web Services by Leonard Richardson (book)
- Baeldung Tutorials on REST APIs (website)
- Practice creating APIs using frameworks like Spring Boot.
- "Spring Boot in Action" by Craig Walls (book)
- Use API testing tools like Postman to test and refine your APIs.
- Postman Documentation (website)
- Learn the basics of REST architecture and HTTP methods (GET, POST, PUT, DELETE).
9. Version Control
Version control involves managing changes to code and collaborating with teams using tools like Git. It ensures code is organized, trackable, and recoverable when needed.
- Learning time
- Takes around 1 to 2 months to become proficient with basic version control workflows and commands.
How to learn:
- Start with Git basics, such as repositories, branches, commits, and merges.
- Pro Git by Scott Chacon (free book)
- GitHub Learning Lab (interactive tutorials)
- Practice collaborating on coding projects using Git and platforms like GitHub.
- Codecademy Git Course (online course)
- Explore advanced concepts like resolving merge conflicts and rebasing.
- Atlassian Git Tutorials (website)
- Start with Git basics, such as repositories, branches, commits, and merges.
10. Security Best Practices
This involves implementing measures to protect applications from vulnerabilities like SQL injection, XSS, and data breaches.
- Learning time
- Can take 2 to 4 months to understand basic security concepts and integrate them into backend systems.
How to learn:
- Study the OWASP Top Ten vulnerabilities and how to mitigate them.
- OWASP Official Website (guidelines)
- "Web Application Security" by Andrew Hoffman (book)
- Practice implementing secure authentication and authorization mechanisms.
- Spring Security Documentation (website)
- Learn encryption techniques and secure data handling practices.
- Java Cryptography Tutorials on Baeldung (website)
- Study the OWASP Top Ten vulnerabilities and how to mitigate them.
11. Performance Optimization
This skill involves improving the efficiency of backend systems to handle higher loads and reduce response times.
- Learning time
- Takes 3 to 6 months, as optimization often requires a combination of theory and hands-on experience.
How to learn:
- Learn about profiling tools to identify bottlenecks in Java applications.
- VisualVM (tool)
- YourKit Java Profiler (tool)
- Study best practices for optimizing code, database queries, and APIs.
- "Java Performance" by Charlie Hunt (book)
- Experiment with caching techniques and load testing tools.
- Apache JMeter Tutorials (tool)
- Learn about profiling tools to identify bottlenecks in Java applications.
12. Testing (Unit and Integration)
Testing ensures the application behaves as expected and reduces the chances of bugs and issues in production.
- Learning time
- Takes around 1 to 3 months to become comfortable with basic testing techniques and frameworks.
How to learn:
- Study the basics of unit and integration testing in Java.
- JUnit 5 User Guide (documentation)
- Practice writing test cases using popular frameworks like JUnit and TestNG.
- "Effective Unit Testing" by Lasse Koskela (book)
- Learn about mocking and test automation.
- Mockito Documentation (website)
- Study the basics of unit and integration testing in Java.
13. Debugging and Troubleshooting
Debugging involves identifying and fixing issues in the code, ensuring smooth application functionality.
- Learning time
- Takes around 1 to 2 months to become proficient with debugging techniques and tools.
How to learn:
- Learn to use IDE debugging tools like breakpoints and watches.
- IntelliJ IDEA Debugger Guide (website)
- Practice identifying issues in sample projects or coding challenges.
- HackerRank Debugging Challenges (platform)
- Study common debugging scenarios and solutions.
- "Practical Debugging in Java" by Peter Fritzson (book)
- Learn to use IDE debugging tools like breakpoints and watches.
14. Cloud Services and Deployment
This involves deploying and managing Java applications on cloud platforms to ensure scalability and reliability.
- Learning time
- Takes around 2 to 4 months to learn basic cloud services and deployment strategies.
How to learn:
- Study the basics of cloud computing and popular platforms like AWS, Azure, or GCP.
- AWS Cloud Practitioner Essentials (course)
- Learn how to containerize applications using Docker and deploy them.
- Docker for Java Developers Tutorials by Baeldung (website)
- Practice deploying a Java application to a cloud platform.
- Heroku Java Deployment Guides (website)
- Study the basics of cloud computing and popular platforms like AWS, Azure, or GCP.
15. Build Tools
Build tools automate tasks like compilation, testing, and deployment, improving development efficiency.
- Learning time
- Takes around 1 to 2 months to master commonly used build tools like Maven and Gradle.
How to learn:
- Learn the basics of setting up and managing projects with build tools.
- Official Maven and Gradle Documentation (website)
- Practice building and managing dependencies for Java projects.
- "Gradle in Action" by Benjamin Muschko (book)
- Watch tutorials on integrating build tools with CI/CD pipelines.
- Pluralsight Maven and Gradle Courses (video course)
- Learn the basics of setting up and managing projects with build tools.
Additional soft skills for Java backend developers
- Communication. IT specialists need to be able to explain complex issues to non-technical team members. This includes articulating the reasoning behind design decisions and collaborating with project managers and stakeholders.
- Documentation. Writing clear and comprehensive documentation for the codebase, APIs, and architecture helps ensure that others can easily understand and maintain the system.
- Collaboration. Working closely with front-end developers, UI/UX designers, product owners, and other stakeholders ensures a seamless integration of the backend with the front end and improves the user experience.
- Creativity. While backend work is typically data-driven, thinking creatively to come up with efficient and scalable solutions for system architecture or performance optimization can make a big difference.
- Analytical thinking. Software development often involves solving complex technical problems. Specialists should be able to approach challenges methodically and logically, breaking down problems into manageable parts.
- Prioritization. IT professionals often juggle multiple tasks, such as bug fixes, feature development, and optimizations. Knowing how to prioritize effectively ensures that critical tasks are completed on time.
- Self-discipline. Whether working in an office or remotely, developers must manage their time independently, ensuring that they meet deadlines without the need for micromanagement.
- Continuous learning. Being open to new programming languages, tools, or frameworks (e.g., moving from Java 8 to 17) and staying updated with industry trends ensures a specialist remains relevant.
- Resilience to change. Whether it’s a change in project requirements or shifts in tech stack, a Java backend developer must be flexible and willing to adapt to new circumstances.
- Conflict resolution. In collaborative environments, disagreements are inevitable. Being able to resolve conflicts diplomatically, whether it's about coding standards, project scope, or timelines, is one of the most important skills required for Java backend developers.
Conclusion
In summary, Java backend developer skills are a blend of technical expertise and problem-solving abilities, which enable developers to craft high-performing and scalable applications.
By focusing on key areas like frameworks, databases, security, and code optimization, developers can significantly enhance their capabilities and contribute to the success of their projects.
Whether you're a novice or an experienced developer, consistently refining these skills will help you stay competitive in the ever-evolving tech industry.
Create your professional Resume in 10 minutes for FREE
Build My Resume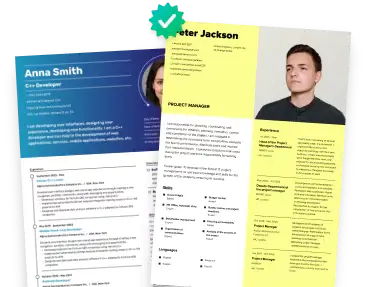